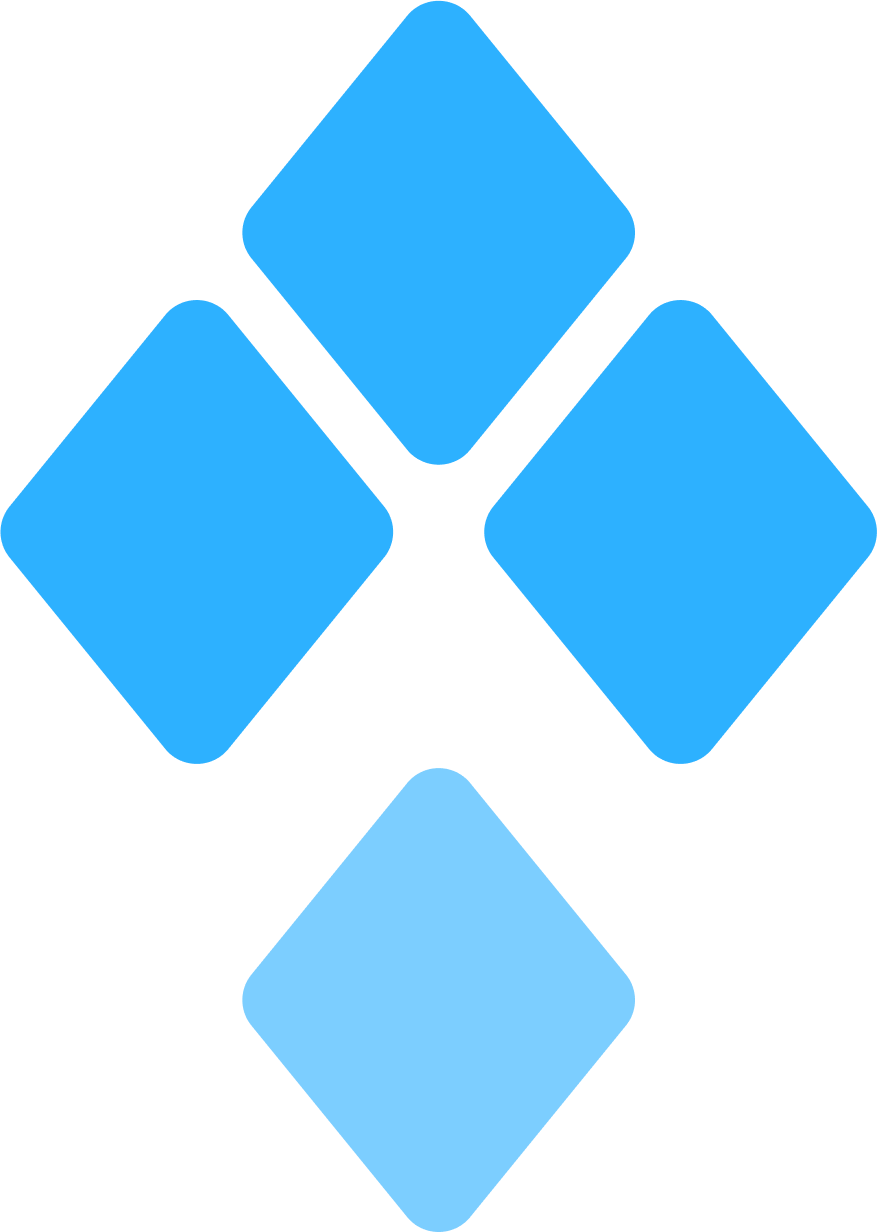
Build the Future of Ethereum Staking
Distributed Validator Technology for secure, decentralized, and scalable staking infrastructure
Decentralized Staking
Secure & Distributed validator operations through DVT technology. Split your validator key across multiple operators for enhanced security and true decentralization.
Developer Tools
Comprehensive SDK and tooling to build the next generation of staking applications. Integrate SSV seamlessly with our developer-first approach.
Enterprise Ready
Production Grade Infrastructure for institutional staking needs. Leverage our battle-tested network of operators with built-in redundancy and monitoring.